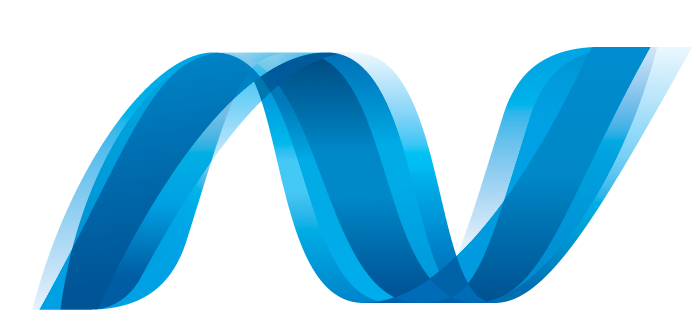
My favorite .NET libraries
Previously I wrote about my favorite JavaScript libraries. If JavaScript libraries appear almost on a daily basis, the case for .NET libraries is different, they appear at a much lower pace and sometimes it's difficult to spot them.
Similar to my previous post, I'll try to stick to open source or free libraries.
EPPLus
From my experience, clients always ask for some sort of processed Excel book. Before Office 2007, Excel manipulation was different. After the Open Office XML Standard, Excel manipulation changed and became somewhat easier. Still, the regular Excel Package library was a bit hard to use.
EPPlus is built over Excel Package and it takes it to a whole new level; creating Excel files directly from .NET is a bliss!
using (ExcelPackage package = new ExcelPackage())
{
ExcelWorksheet workSheet = package.Workbook.Worksheets.Add("My Cool Excel Page");
// You can load data directly from a DataTable, a Collection and other options!
workSheet.Cells["A1"].LoadFromDataTable(myDataTable, true);
Response.ContentType = "application/vnd.openxmlformats-officedocument.spreadsheetml.sheet";
Response.AddHeader("content-disposition", "attachment; filename=ExcelDemo.xlsx");
Response.BinaryWrite(package.GetAsByteArray());
}
Be sure to check the documentation. You can even insert grahics, macros and formulas directly from your code!
iTextSharp
Similar to Excel books, PDF files are often a common client requirement. iTextSharp is incredibly robust, and allow all sorts of PDF manipulation.
One of the most interesting features is that it allows digital signatures, a very complex task now made easy. Be sure to check it out and also PDF Sharp, which is also incredibly good.
Dynamic LINQ
The first time I used LINQ I fell in love. LINQ is a powerful integrated query language compatible with C#, Visual Basic and other .NET languages. LINQ allows you to query over collections, SQL databases and many other things.
// Sample LINQ query
int[] numbers = { 5, 4, 1, 3, 9, 8, 6, 7, 2, 0 };
var lowNums = from n in numbers
where n < 5
select n;
LINQ allows where clausing, aggregate features and many other things. The only "problem" with LINQ is that you need a compile-time knowledge of the data model, Dynamic LINQ on the other hand takes LINQ queries to a whole new level, it is brutal.
using System.Linq.Dynamic; //Import the Dynamic LINQ library
// Standard LINQ query
var result = myQuery
.Where(x => x.Name == "Santiago")
.Select(x => new { x.Name, x.LastName });
// Dynamic LINQ way, which lets you do the same thing
// without knowing the data model beforehand
var result = myQuery
.Where("Name=\"Santiago\"")
.Select("new (Name, LastName)");
Extend
Extend is a set of extension methods for several .NET's objects, such as String, Date, Array and many more. Incredible useful.
var list = new List<String>();
// From here, these are extension methods for a collection
list.AddIf(x => new Random().Next(0, 10) % 2 == 0, "Value");
list.AddIfNotContains( "Value #1");
list.AddRange("Value #1", "Value #2", "Value #3");
list.AddRangeIfNotContains("Value #1", "Value #2", "Value #3");
list.AddRangeIf(x => new Random().Next(0, 10) % 2 == 0, "Value", "Value #2", "Value #3");
Be sure to check the project's page.