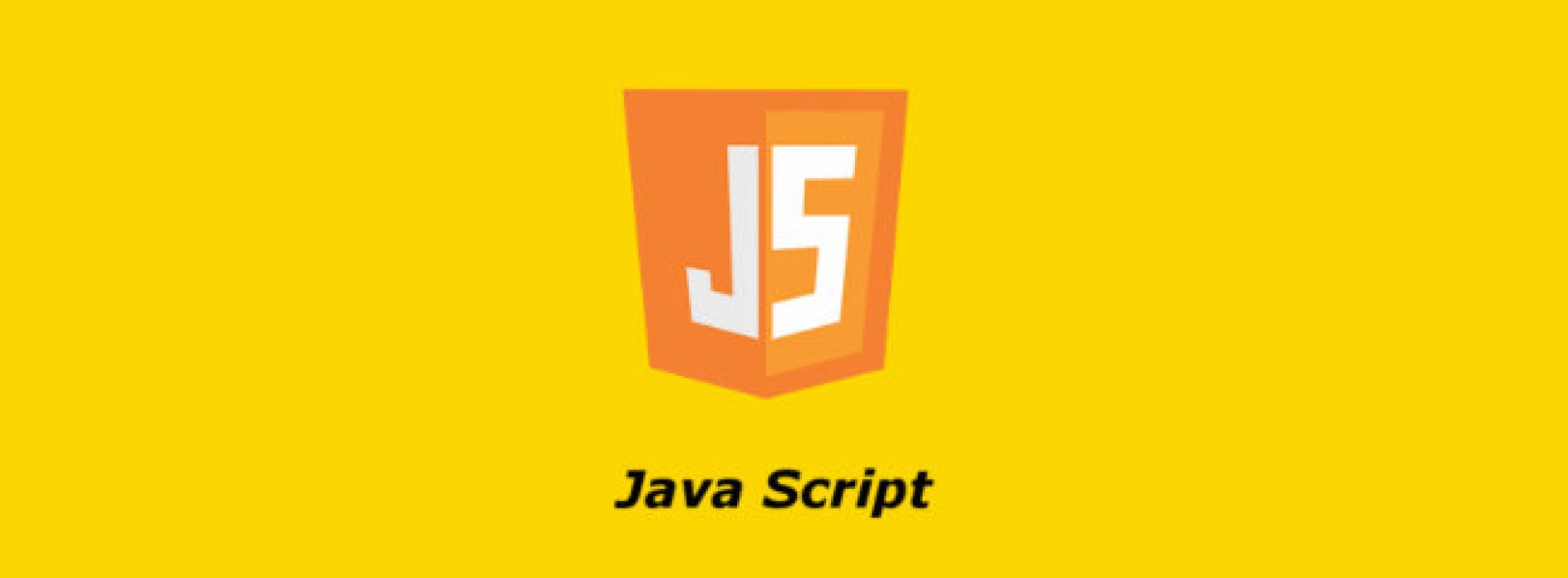
Securing values in JavaScript
Using global variables in JavaScript is not commonly considered a good practice.
Besides these constraints, in web development, every user has access to the Window (or global) object. By putting variables on the global scope, you give any user the ability to see or change your variables.
So what can you do?
Well, if using globals is necessary in your application, you must be sure to make it as secure as possible.
Design Patterns
In JavaScript there are many design patterns you can consider according to your application's needs.
So far, I feel very confident with the module pattern. It supports a clean approach with private data, among many other things, such as global imports.
Anonymous closures are one of my favorite things about this pattern!
(function () {
// All variables and functions are in this scope only
// Still maintains access to all globals
function myFunction1() {
someOtherFunction();
}
}());
function someOtherFunction() {
// I don't get access to any of the above :(
}
With this approach, you can tighten your application's security.
Closures are a feature of JavaScript that allows us to implement data hiding which is roughly equivalent to private variables in languages like C++ or Java.
What if you wanted to permanently lock a certain value?
Constants
The EC2015 language specification supports constants.
const MY_CONSTANT = "some-sexy-value";
The only downside? They don't work in IE 8, IE 9 and IE 10.
EDIT 13/03/2017: A lot has happened since I first wrote this article. The correct and "modern" way to do variable scoping is through let and const, var should be avoided. The bellow approach is actually a closure.
My Approach
(function () {
var lockedValues = (function () {
var privateOption = {
'SEXYMESSAGE': getSexyMessage()
};
return {
get: function (property) {
return privateOption[property];
}
};
function getSexyMessage() {
return "Hey, there!";
}
})();
function letsDoStuff() {
alert(lockedValues.get("SEXYMESSAGE"));
}
}());
Once the letsDoStuff() function is called it will print our sexy message. What is interesting is that this approach mimics constant values, they can not be modified!