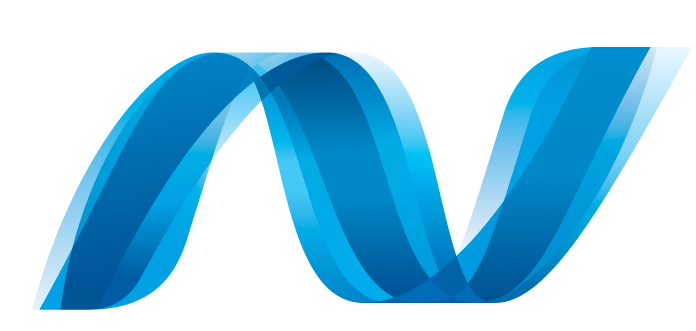
ASP.NET interview questions
This is a collection of questions that I have been asked for some jobs that include ASP.NET development. There are many web pages that contain a similar set of questions, these just are a personal recolection.
The topic for these questions not only refers to the .NET framework, but also several aspects that can be related to it.
What's boxing and unboxing?
It's the process of converting a value to an object or any interface type implemented by this value type.
When the CLR boxes a value type, it wraps the value inside a System.Object and stores it on the managed heap.
Unboxing extracts the value type from the object. Boxing is implicit; unboxing is explicit.
int i = 123;
// The following line boxes i.
object o = i;
o = 123;
// The following line unboxes o.
i = (int)o;
What's an extension method?
Extension methods enables you to "add" methods to existing types, without creating a new derived type, recompiling, or otherwise modifying the original type.
Extension methods are a special kind of static method, but they are called as if they were instance methods on the extended type.
For example, we want to know if a certain string is a number or not. We can extend the String class to achieve this. It's important to define a static class with a set of static methods.
public static class MyExtensionMethods
{
// The "this" word tells the compiler that this is an extension method for the String class
public static bool IsNumeric(this string s)
{
float output;
return float.TryParse(s, out output);
}
}
In order to use it, you can do this:
string test = "42";
if (test.IsNumeric())
{
Console.WriteLine("Yup");
}
else
{
Console.WriteLine("Nope");
}
What's a delegate?
A delegate allows you to encapsulate a reference to a method inside a delegate object. The delegate object can then be used like any other object, and can be passed to code which can call the referenced method.
Binding Events to Event Handlers is usually your first introduction to delegates in .Net.
// This is how you declare a Delegate
public delegate void MyDelegate(string message);
// Create a method for a delegate.
public static void DelegateMethod(string message)
{
System.Console.WriteLine(message);
}
// Instantiate the delegate.
MyDelegate mySexyHandler = DelegateMethod;
// Call the delegate.
mySexyHandler("Hello World");
What's an interface?
An interface is defined as a syntactical "contract" that all the classes inheriting the interface should follow.
public interface IOrders
{
// Interface members
void ShowTransaction();
double GetTotal();
}
A class inherting from IOrders would need the methods ShowTransaction() and GetTotal() in order to compile.
Interfaces define properties, methods, and events, which are the members of the interface. Interfaces contain only the declaration of the members.
What's CLR?
The Common Language Runtime (CLR) is the virtual machine component of Microsoft's .NET framework, it manages the execution of .NET programs.
A process known as just-in-time compilation converts compiled code into machine instructions which the computer's CPU then executes.
What's CLI?
Part of Microsoft's .NET strategy, Common Language Infrastructure (CLI) enables an application program written in any of several commonly-used programming languages to be run on any operating system using a common runtime program rather than a language-specific one.
Common Language Infrastructure provides a virtual execution environment comparable to the one provided by Sun Microsystems for Java programs. In both environments, CLI and Java use a compiler to process language statements (also known as source code) into a preliminary form of executable code called bytecode.
What's the difference between public, private and protected method?
Called "Access Modifiers", these words determine what part of the code can be accessed in your assembly or other assembly.
public: The type or member can be accessed by any other code in the same assembly or another assembly that references it.
private: The type or member can be accessed only by code in the same class or struct.
protected: The type or member can be accessed only by code in the same class or struct, or in a class that is derived from that class.
What's MSIL?
MSIL is the former name for what is now known as Common Intermediate Language, it is the lowest-level human-readable programming language defined by the CLI (see above) specification. Languages which target a CLI-compatible runtime environment compile to CIL.
What's a struct in C#?
A struct is a data structure which defines a collection of objects and methods.
Like an entry in the phone book of a cellphone, each instance of the struct contains the same pieces of information (such as the person’s name, telephone number, and email address).
The key question is in which situations a struct has benefits over a class, since they are very similar.
public struct PhoneBook
{
public String name;
public String telephone;
public String email;
}
The properties of a struct cannot have initializers, unless those properties are marked as const or static.
Unlike a class, you do not always need to use the keyword new to use a struct. Structs can be declared like simple data types:
PhoneBook santi;
At this point, the object is ready to use:
PhoneBook santi;
santi.name = "Santiago";
santi.email = "myawesomeemail@me.com";
Does C# allow multiple inheritance?
No, but it does allow multiple inheritance from interfaces.
What's the purpose of the Dispose method.
To cleanup the memory of unmanaged resources. The Dispose method generally doesn't free managed memory—typically, it's used for early reclamation of only the unmanaged resources to which a class is holding references. In other words, this method can release the unmanaged resources in a deterministic fashion.
What does the keyword "using" accomplish?
The reason for the "using" statement is to ensure that the object is disposed as soon as it goes out of scope, and it doesn't require explicit code to ensure that this happens.
What are generations? List and describe them
While memory allocation on the managed heap is fast, Garbage Collector itself may take some time. With this in mind several optimisations have been made to improve performance. The Garbage Collector supports the concept of generations, based on the assumption that the longer an object has been on the heap, the longer it will probably stay there.
Generation 0 identifies a newly created object that has never been marked for collection.
Generation 1 identifies an object that has survived a GC (marked for collection but not removed because there was sufficient heap space).
Generation 2 identifies an object that has survived more than one sweep of the GC.
Give three conditions for a .Net Garbage Collection?
Garbage collection occurs when one of the following conditions is true:
The system has low physical memory.
The memory that is used by allocated objects on the managed heap surpasses an acceptable threshold. This threshold is continuously adjusted as the process runs.
The GC.Collect method is called. In almost all cases, you do not have to call this method, because the garbage collector runs continuously. This method is primarily used for unique situations and testing.
Explain the purpose and advantage of .NET Garbage Collection
- Allow us to develop an application without having worry to free memory.
- Allocates memory for objects efficiently on the managed heap.
- Reclaims the memory for no longer used objects and keeps the free memory for future allocations.
- Provides memory safety by making sure that an object cannot use the content of another object.
Bibliography
"Boxing and Unboxing (C# Programming Guide)." Boxing and Unboxing (C# Programming Guide). Microsoft, n.d. Web. 28 July 2015. https://msdn.microsoft.com/en-us/library/yz2be5wk.aspx.
"Extension Methods (C# Programming Guide)." Extension Methods (C# Programming Guide). Microsoft, n.d. Web. 28 July 2015. https://msdn.microsoft.com/en-us/library/bb383977.aspx.
"Delegates Tutorial." (C#). Microsoft, n.d. Web. 28 July 2015. https://msdn.microsoft.com/en-us/library/aa288459(v=vs.71).aspx.
"What Is Common Language Infrastructure (CLI)? - Definition from WhatIs.com." SearchSOA. Margaret Rouse, June 2007. Web. 27 Aug. 2015. http://searchsoa.techtarget.com/definition/Common-Language-Infrastructure.
"Access Modifiers (C# Programming Guide)." Access Modifiers (C# Programming Guide). Microsoft, n.d. Web. 27 Aug. 2015. https://msdn.microsoft.com/en-us/library/ms173121.aspx.
"InterviewCity.com." What Is Intermediate Language(IL)/MSIL/CIL in .NET. N.p., 13 Apr. 2010. Web. 27 Aug. 2015. http://www.interviewcity.com/2010/04/what-is-intermediate-languageilmsilcil.html.
"Using Structs in C#: How and Why?" Udemy Blog. Jennifer Marsh, 22 Apr. 2014. Web. 28 Aug. 2015. https://blog.udemy.com/c-sharp-struct/.
"When and How to Use Dispose and Finalize in C#." When and How to Use Dispose and Finalize in C#. Joydip Kanjilal, 27 Nov. 2006. Web. 28 Aug. 2015. http://www.devx.com/dotnet/Article/33167.
"Garbage Collection in .NET." CodeProject. Chris Maunder, 17 June 2002. Web. 28 Aug. 2015. http://www.codeproject.com/Articles/1060/Garbage-Collection-in-NET.